Random、NumPy、SciPy
前回、Pythonのmatplotlibでギリシャ文字を表示する方法を紹介しました。
あわせて読みたい
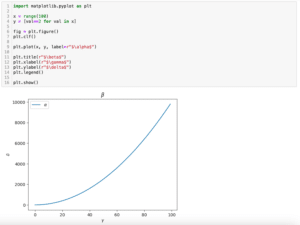
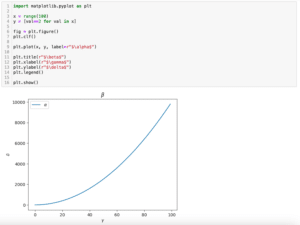
【matplotlib】ギリシャ文字を表示する方法[Python]
matplotlib 前回、Pythonのmatplotlibで斜体(イタリック)文字、上付き文字、下付き文字を使う方法を紹介しました。 今回はmatplotlibでギリシャ文字を表示する方法を…
今回はRandomモジュール、NumPy、SciPyの3種で正規分布(ガウス分布)に従うランダムな値を取得する方法を紹介します。
それでは始めていきましょう。
Randomモジュールの場合
Randomモジュールを用いる場合、「random.gauss(mu, sigma)」でで正規分布に従うランダムな値を取得できます。
import random
print(random.gauss(0, 1))
実行結果
-1.5371829296125312
一つの値だけでは正規分布に従っているか分からないので、たくさん取得し、ヒストグラムを描いてみます。
import random
import matplotlib.pyplot as plt
x = range(1000000)
y = [random.gauss(0, 1) for _ in x]
fig = plt.figure()
plt.clf()
plt.hist(y, bins=100)
plt.show()
実行結果
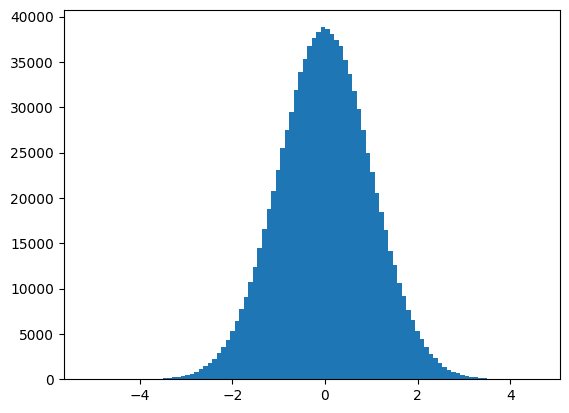
ちなみに「random.normalvariate(mu, sigma)」でも正規分布に従うランダムな値を取得することができます。
import random
print(random.normalvariate(0, 1))
実行結果
0.8381527170880502
import random
import matplotlib.pyplot as plt
x = range(1000000)
y = [random.normalvariate(0, 1) for _ in x]
fig = plt.figure()
plt.clf()
plt.hist(y, bins=100)
plt.show()
実行結果
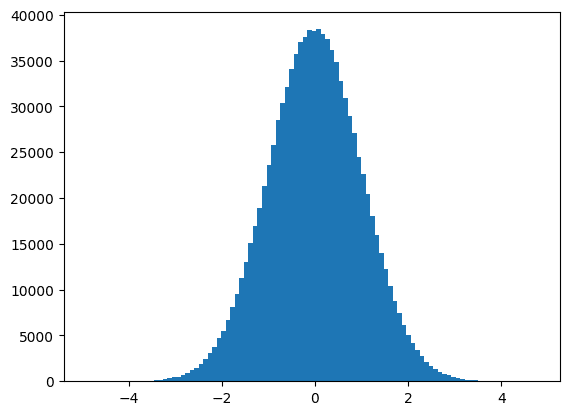
NumPyの場合
NumPyの場合はまず「rng = np.random.default_rng()」でジェネレータのインスタンスを生成し、「rng.normal(mu, sigma, size)」でランダムな値のリストを生成します。
import numpy as np
rng = np.random.default_rng()
print(rng.normal(0, 1, 5))
実行結果
[ 1.14280621 -0.90061618 0.66710664 -1.13582515 -2.22449659]
先ほどの「random.gauss()」とは異なり、直接複数の値をリストとして作成することができます。
import numpy as np
import matplotlib.pyplot as plt
x = range(1000000)
rng = np.random.default_rng()
y = rng.normal(0, 1, len(x))
fig = plt.figure()
plt.clf()
plt.hist(y, bins=100)
plt.show()
実行結果
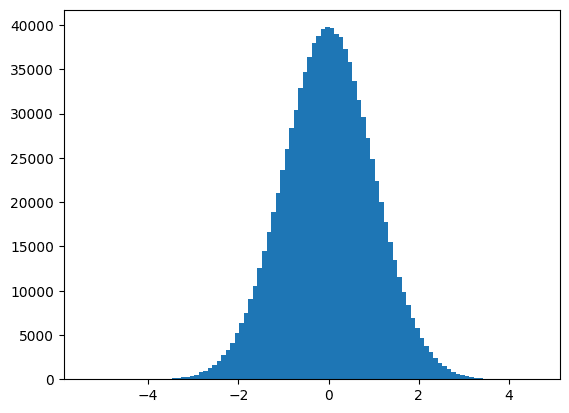
SciPyの場合
SciPyの場合は「scipyのstats」をインポートし、「stats.norm.rvs(mu, sigma, size)」で正規分布に従うランダムな値のリストを作成できます。
from scipy import stats
print(stats.norm.rvs(0, 1, 5))
実行結果
[-0.40377819 -0.23260934 0.32406025 0.39979899 0.49024652]
from scipy import stats
import matplotlib.pyplot as plt
x = range(1000000)
y = stats.norm.rvs(0, 1, len(x))
fig = plt.figure()
plt.clf()
plt.hist(y, bins=100)
plt.show()
実行結果
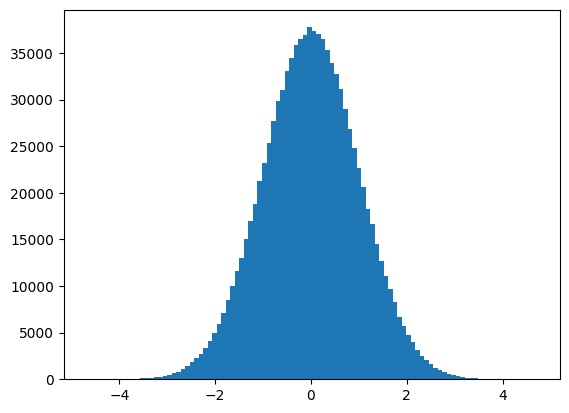
今回は正規分布(ガウス分布)に従うランダムな値の取得方法を紹介しましたが、それぞれのライブラリにおいて他の分布に従うランダムな値の取得ができる関数が存在します。
もし気になったら調べてみてください。
次回はNumPyでジェネレータを使ったランダムな数値の取得方法を紹介します。
あわせて読みたい
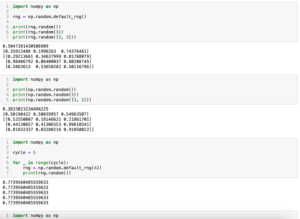
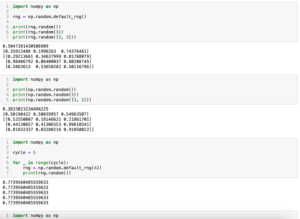
【NumPy】ジェネレータを使ったランダムな数値の取得方法の紹介と使わない方法との比較[Python]
NumPy 前回、PythonのNumPyとSciPyで正規分布(ガウス分布)に従うランダムな値を取得する方法を紹介しました。 今回はNumPyでジェネレータを使ったランダムな数値の取…
ではでは今回はこんな感じで。
コメント