matplotlib
前回、Pythonのmatplotlibでガウス分布、ラプラス分布、ローレンツ分布(コーシー分布)を自作関数化してグラフ表示する方法を紹介しました。
あわせて読みたい
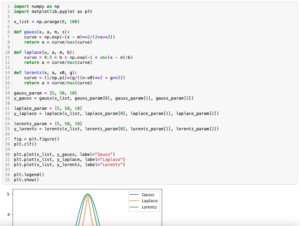
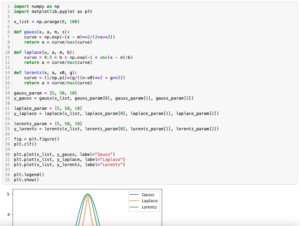
【matplotlib】ガウス分布、ラプラス分布、ローレンツ分布(コーシー分布)を自作関数化してグラフ表示…
matplotlib 前回、PythonのScikit-learnで二つのグラフの一致度を確認する方法を紹介しました。 今回はガウス分布、ラプラス分布、ローレンツ分布を自作関数化してグラ…
今回はmatplotlibでピークを境に左右の形状が非対称な分布を作成する方法を紹介します。
前回紹介したガウス分布の作成方法はこんな感じでした。
import numpy as np
import matplotlib.pyplot as plt
x_list = np.arange(0, 100)
def gauss(x, a, m, s):
curve = np.exp(-(x - m)**2/(2*s**2))
return a * curve/max(curve)
gauss_param = [5, 50, 10]
y_gauss = gauss(x_list, gauss_param[0], gauss_param[1], gauss_param[2])
fig = plt.figure()
plt.clf()
plt.plot(x_list, y_gauss)
plt.show()
実行結果
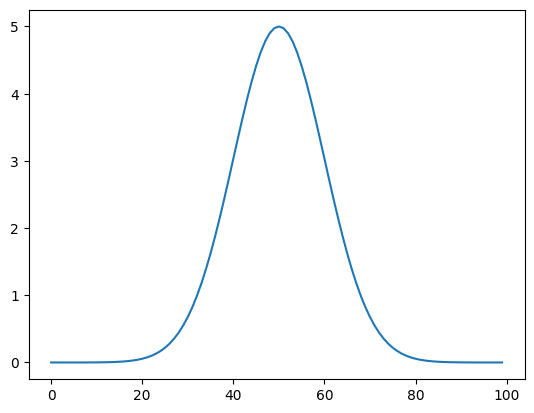
これを元に左右非対称の分布を作成していきます。
それでは始めていきましょう。
ピークを境に左右非対称な分布
ピークを境に左右非対称な分布を作成する方法として、ピークを境に分布を変えてしまうことが考えられます。
ということでまずは2つの異なる分布を用意し、それぞれをピーク位置で分割し、分割したもののうち最終的に使いたいものだけ結合させるということをしてみます。
import numpy as np
import matplotlib.pyplot as plt
x_list = np.arange(0, 100)
def gauss(x, a, m, s):
curve = np.exp(-(x - m)**2/(2*s**2))
return a * curve/max(curve)
a = 5
m = 50
gauss_param1 = [a, m, 10]
gauss_param2 = [a, m, 5]
y_gauss1 = gauss(x_list, gauss_param1[0], gauss_param1[1], gauss_param1[2])
y_gauss2 = gauss(x_list, gauss_param2[0], gauss_param2[1], gauss_param2[2])
y_gauss12 = np.concatenate([y_gauss1[:m], y_gauss2[m:]])
fig = plt.figure()
plt.clf()
plt.plot(x_list, y_gauss12)
plt.show()
実行結果
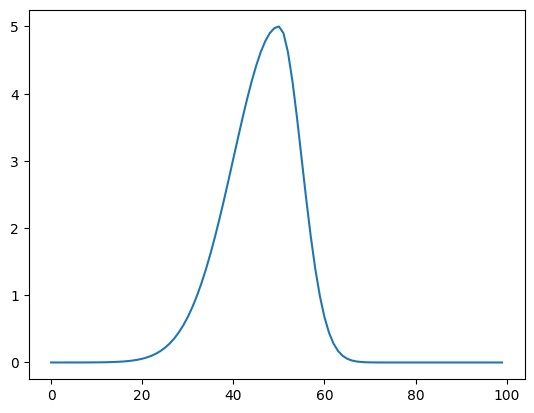
今回は左右に異なるガウス分布をもつ分布を作成してみましたが、この方法を使えば左右にガウス分布とラプラス分布をもつ分布を作成することもできます。
ということで自作関数化したものがこちらです。
import numpy as np
import matplotlib.pyplot as plt
x_list = np.arange(0, 100)
a = 5
m = 50
s1 = 10
s2 = 5
s = 10
b = 10
def gauss(x, a, m, s):
curve = np.exp(-(x - m)**2/(2*s**2))
return a * curve/max(curve)
def doublegauss(x, a, m, s1, s2):
y_gauss1 = gauss(x, a, m, s1)
y_gauss2 = gauss(x, a, m, s2)
y_doublegauss = np.concatenate([y_gauss1[:m], y_gauss2[m:]])
return y_doublegauss
y_doublegauss = doublegauss(x_list, a, m, s1, s2)
fig = plt.figure()
plt.clf()
plt.plot(x_list, y_doublegauss)
plt.show()
実行結果
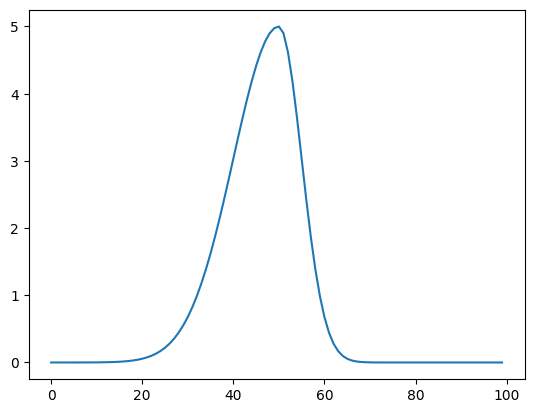
数学的に正しいのかまでは保証できませんが、簡易的にピークフィッティングなんかに使うのは大丈夫だと思います。
次回はガウス分布とローレンツ分布を合わせた関数(フォークト関数)、そしてその左右非対称化をした分布の作成方法を紹介します。
あわせて読みたい
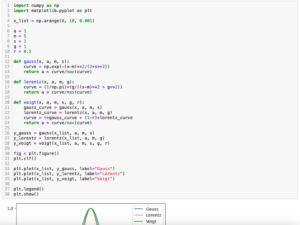
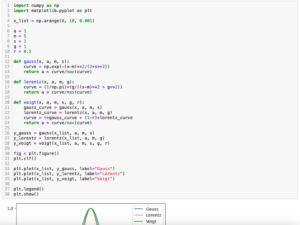
【matplotlib】ガウス分布とローレンツ分布を合わせたフォークト関数(voigt)の作成方法と左右非対称化の…
matplotlib 前回、Pythonのmatplotlibでピークを境に左右の形状が非対称な分布の作成方法を紹介しました。 今回はガウス分布とローレンツ分布を合わせたフォークト関数…
ではでは今回はこんな感じで。
コメント