NumPy
前回、PythonのSciPyでargrelmax、argrelminを使って極大値、極小値を取得する方法を紹介しました。
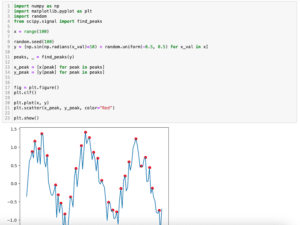
今回はNumPyのndarrayではインデックスをまとめたリストで直接要素を取得可能であることを紹介します。
それでは始めていきましょう。
通常のリストの場合
通常のリストの場合、インデックスを指定してリスト内の要素を取得するには「[](角括弧)」内に1つのインデックスを入れて指定します。
a = ["a", "b", "c", "d", "e"]
print(a[2])
実行結果
c
その際、インデックスだけをまとめたリストがあったとしても、もう一つのリスト(ここではリストa)から直接使用して要素を取得することはできません。
a = ["a", "b", "c", "d", "e"]
b = [1, 2, 4]
print(a[b])
実行結果
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
Cell In[16], line 4
1 a = ["a", "b", "c", "d", "e"]
2 b = [1, 2, 4]
----> 4 print(a[b])
TypeError: list indices must be integers or slices, not list
スライスを用いて、インデックス用の要素を取得したとしてももう一つのリスト(リストa)の要素を取得することはできません。
a = ["a", "b", "c", "d", "e"]
b = [1, 2, 4]
print(a[b[0:2]])
実行結果
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
Cell In[8], line 4
1 a = ["a", "b", "c", "d", "e"]
2 b = [1, 2, 4]
----> 4 print(a[b[0:2]])
TypeError: list indices must be integers or slices, not list
そのためfor文を使ってインデックス用のリストから要素を一つずつ取り出して、もう一つのリスト(リストa)から要素を取得する必要があります。
a = ["a", "b", "c", "d", "e"]
b = [1, 2, 4]
for ind in b:
print(a[ind])
実行結果
b
c
e
もし取り出した要素でリストにまとめるのであれば、上の例と同様にfor文を使ってインデックスを取り出し、要素を取り出すか、リスト内包表記で要素をまとめるといった手順が必要になります。
a = ["a", "b", "c", "d", "e"]
b = [1, 2, 4]
c = []
for ind in b:
c.append(a[ind])
d = [a[ind] for ind in b]
print(c)
print(d)
実行結果
['b', 'c', 'e']
['b', 'c', 'e']
NumPyのndarrayの場合
NumPyのndarrayの場合、インデックス用のリストをそのままインデックスとして使用することができます。
import numpy as np
a = np.array(["a", "b", "c", "d", "e"])
b = [1, 2, 4]
print(a[b])
実行結果
['b' 'c' 'e']
両方ともNumPyのndarrayでも大丈夫です。
import numpy as np
a = np.array(["a", "b", "c", "d", "e"])
b = np.array([1, 2, 4])
print(a[b])
実行結果
['b' 'c' 'e']
取得したい要素をまとめたリストがNumPyのndarrayでない場合(インデックス側のリストがNumPyのnadarrayでも)はエラーとなります。
import numpy as np
a = ["a", "b", "c", "d", "e"]
b = np.array([1, 2, 4])
print(a[b])
実行結果
---------------------------------------------------------------------------
TypeError Traceback (most recent call last)
Cell In[12], line 6
3 a = ["a", "b", "c", "d", "e"]
4 b = np.array([1, 2, 4])
----> 6 print(a[b])
TypeError: only integer scalar arrays can be converted to a scalar index
ちなみにインデックス用のリストは二次元リストでも三次元リストでも使用可能です。
import numpy as np
a = np.array(["a", "b", "c", "d", "e"])
b = [[1, 2, 4],[0, 1, 2]]
print(a[b])
実行結果
[['b' 'c' 'e']
['a' 'b' 'c']]
import numpy as np
a = np.array(["a", "b", "c", "d", "e"])
b = [[[1, 2, 4],[0, 1, 2]],[[4, 3, 2],[2, 1, 0]]]
print(a[b])
実行結果
[[['b' 'c' 'e']
['a' 'b' 'c']]
[['e' 'd' 'c']
['c' 'b' 'a']]]
今まで通常のリストでfor文やリスト内包表記で一つずつ取得していたことを思うと結構便利なので覚えておくといいでしょう。
次回はmatplotlibのpcolormeshで二次元カラープロットを表示する方法を紹介します。
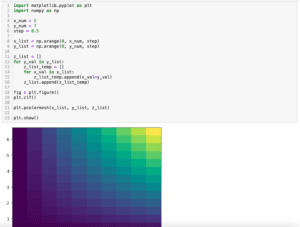
ではでは今回はこんな感じで。
コメント