tqdmモジュール
前回、Pythonで再帰処理を使って全組合せを作ってみるというのを紹介しました。
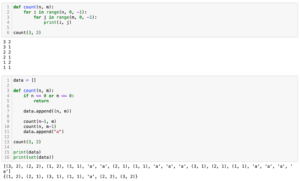
今回はtqdmモジュールというものを使って、繰り返し処理の進捗具合をプログレスバーとして表示する方法を紹介します。
それでは始めていきましょう。
インストール
まずはtqdmモジュールをインストールします。
インストールはいつものようにpipで行います。
pip install tqdm
tqdmモジュールの基本
tqdmモジュールは「tqdm(リストなど)」としてリストなど繰り返すオブジェクトを格納したのち、for文で繰り返し処理することで使用することができます。
つまりこんな感じ。
from tqdm import tqdm
import time
repeat = tqdm(range(10))
for i in repeat:
time.sleep(1)
range関数を使わず、リストを直接入れても大丈夫です(結果は上と同じなので割愛します)。
from tqdm import tqdm
import time
ls = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9]
repeat = tqdm(ls)
for i in repeat:
time.sleep(1)
タプルでも辞書でもSet型でも大丈夫です(結果は上と同じなので割愛します)。
from tqdm import tqdm
import time
tup = (0, 1, 2, 3, 4, 5, 6, 7, 8, 9)
repeat = tqdm(ls)
for i in repeat:
time.sleep(1)
from tqdm import tqdm
import time
dic = {"a":0, "b":1, "c":2, "d":3, "e":4, "f":5, "g":6, "h":7, "i":8, "j":9}
repeat = tqdm(dic)
for i in repeat:
time.sleep(1)
from tqdm import tqdm
import time
st = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9}
repeat = tqdm(ls)
for i in repeat:
time.sleep(1)
また今回はわかりやすいようにまず変数に格納していますが、直接for文に書いてしまっても大丈夫です(結果は上と同じなので割愛します)。
from tqdm import tqdm
import time
for i in tqdm(range(10)):
time.sleep(1)
進捗を手動で設定する
上記の場合はリストやタプルの長さから進捗度合いを自動で設定していますが、手動で設定することも可能です。
その場合は「変数 = tqdm(total=数値)」で進捗のステップ数を設定し、「変数.update(数値)」で進捗度合いを進めることができます。
from tqdm import tqdm
import time
progress = tqdm(total = 100)
for i in range(10):
progress.update(5)
time.sleep(1)
ただしこの際、totalの値とupdateで進められた進捗度合いが合わないと途中で止まってしまったり、表示が消えてしまったりするので注意です。
from tqdm import tqdm
import time
progress = tqdm(total = 100)
for i in range(10):
progress.update(5)
time.sleep(1)
実行結果
50%|█████████████████████ | 50/100 [00:09<00:09, 5.07it/s]
from tqdm import tqdm
import time
progress = tqdm(total = 100)
for i in range(10):
progress.update(15)
time.sleep(1)
実行結果
150it [00:19, 15.22it/s]
自作関数で使う場合の注意
自作関数でtqdmモジュールを使う場合、中に繰り返し処理があるからと言って、関数に対してtqdmモジュールを使っても正しく動作しません。
from tqdm import tqdm
import time
def test(n):
for i in range(n):
time.sleep(1)
tqdm(test(10))
実行結果
0it [00:00, ?it/s]
<tqdm.std.tqdm at 0x1123c7a00>
あくまでも繰り返すためのオブジェクトに対してtqdmモジュールを使います。
from tqdm import tqdm
import time
def test(l):
for i in l:
time.sleep(1)
test(tqdm(range(10)))
実行結果
100%|███████████████████████████████████████████| 10/10 [00:10<00:00, 1.00s/it]
処理の概要を表示
tqdmのオプションに「desc=”処理の概要”」を追加することで、プログレスバーの最初に処理の概要を表示することができます。
from tqdm import tqdm
import time
def test(l):
for i in l:
time.sleep(1)
l = range(10)
test(tqdm(l, desc="test_program"))
実行結果
test_program: 100%|█████████████████████████████| 10/10 [00:10<00:00, 1.01s/it]
時間のかかる処理を行う場合、どれくらい進んでいるかを見ることでそのまま続けるか、他のやり方に変えるか判断できますし、何よりいつまでかかるんだろうと不安になるストレスが減るので積極的に使っていきたいものですね。
次回はPythonでカレンダーを表示するcalenderモジュールを紹介します。
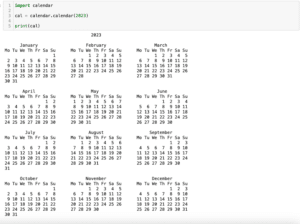
ではでは今回はこんな感じで。
コメント