JQuery
前回、JQuery(JavaScript)で数値と文字列の計算に関して紹介しました。
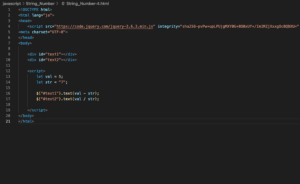
今回はボタンや入力欄、ドロップダウンメニューなどを選択不可(入力不可)にする方法を紹介します。
選択可能か状態を取得する方法
まずはJQueryでボタンやドロップダウンメニュー、入力欄が選択可能か状態を取得する方法です。
ここではボタンを例に解説しますが、入力欄やドロップダウンメニュー、ラジオボタンなどでも同じです。
「let 変数 = $(“#セレクタ”).prop(“disabled”)」でそのボタンが押せるか(ドロップダウンメニューなら選択可能か)の状態が取得できます。
<!DOCTYPE html>
<html lang="ja">
<head>
<script src="https://code.jquery.com/jquery-3.6.3.min.js" integrity="sha256-pvPw+upLPUjgMXY0G+8O0xUf+/Im1MZjXxxgOcBQBXU=" crossorigin="anonymous"></script>
<meta charset="UTF-8">
</head>
<body>
<p><button id="button">Button</button></p>
<p><button id="b1">b1_button</button></p>
<script>
$("#button").on("click", function(){
let b1_check = $("#b1").prop("disabled");
console.log(b1_check);
})
</script>
</body>
</html>
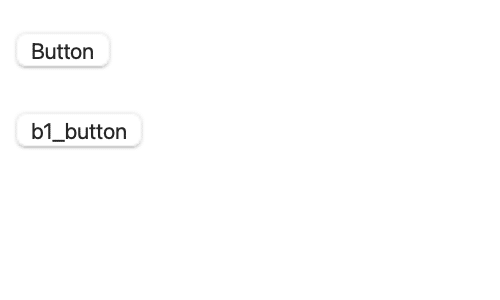
これで上のボタン(IDが”button”)を押すたびに、下のボタン(IDが”b1”)の状態を取得できます。
ちなみにこの状態では”b1”のボタンは押せる状態ですので「true」がコンソールに表示されます。
ボタンを押せない(または選択不可)にする方法
次にボタンを押せない、ドロップダウンメニューを選択できない、入力欄に入力できない状態にする方法です。
それぞれのhtmlタグに「disabled=”disabled”」を追加します。
<!DOCTYPE html>
<html lang="ja">
<head>
<script src="https://code.jquery.com/jquery-3.6.3.min.js" integrity="sha256-pvPw+upLPUjgMXY0G+8O0xUf+/Im1MZjXxxgOcBQBXU=" crossorigin="anonymous"></script>
<meta charset="UTF-8">
</head>
<body>
<p><button id="button">Button</button></p>
<p><button id="b1" disabled="disabled">b1_button</button></p>
<script>
$("#button").on("click", function(){
let b1_check = $("#b1").prop("disabled");
console.log(b1_check);
})
</script>
</body>
</html>
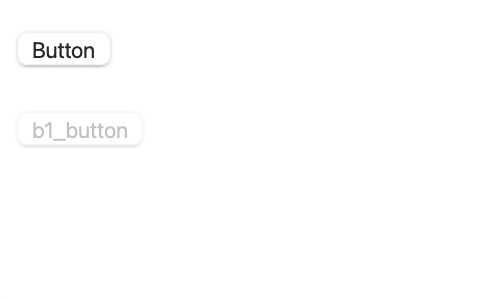
これで上のボタン(IDが”button”)を押すと下のボタン(IDが”b1”)の状態として「false」が返ってきます。
ただ何をしても下のボタンは押せません。
条件によってボタンを状態を変える方法
次に条件によってボタンを押せたり、押せなかったりを変える方法を紹介します。
先ほどボタンの状態を「$(“#セレクタ”).prop(“disabled”)」で取得しましたが、さらに「$(“#セレクタ”).prop(“disabled”, false)」にするとボタンを押せる状態に、「$(“#セレクタ”).prop(“disabled”, true)」にするとボタンが押せない状態に変更することができます。
先ほどの上のボタン(IDが”button”)を押すと、下のボタン(IDが”b1″)の状態を変更するようにしてみましょう。
<!DOCTYPE html>
<html lang="ja">
<head>
<script src="https://code.jquery.com/jquery-3.6.3.min.js" integrity="sha256-pvPw+upLPUjgMXY0G+8O0xUf+/Im1MZjXxxgOcBQBXU=" crossorigin="anonymous"></script>
<meta charset="UTF-8">
</head>
<body>
<p><button id="button">Button</button></p>
<p><button id="b1">b1_button</button></p>
<script>
$("#button").on("click", function(){
let b1_check = $("#b1").prop("disabled");
if(b1_check==true){
$("#b1").prop("disabled", false);
}else if(b1_check==false){
$("#b1").prop("disabled", true);
}
})
</script>
</body>
</html>
入力欄を入力可能/不可能にする方法
入力欄を入力可能/不可能にする方法も「$(“#セレクタ”).prop(“disabled”, false)」と「$(“#セレクタ”).prop(“disabled”, true)」を使います。
<!DOCTYPE html>
<html lang="ja">
<head>
<script src="https://code.jquery.com/jquery-3.6.3.min.js" integrity="sha256-pvPw+upLPUjgMXY0G+8O0xUf+/Im1MZjXxxgOcBQBXU=" crossorigin="anonymous"></script>
<meta charset="UTF-8">
</head>
<body>
<p><button id="button">Button</button></p>
<p><input id="i1"></p>
<script>
$("#button").on("click", function(){
let i1_check = $("#i1").prop("disabled");
if(i1_check==true){
$("#i1").prop("disabled", false);
}else if(i1_check==false){
$("#i1").prop("disabled", true);
}
})
</script>
</body>
</html>
ドロップダウンメニューを選択可能/不可能にする方法
次にドロップダウンメニューを選択可能/不可能にする方法ですが、こちらも同じく「$(“#セレクタ”).prop(“disabled”, false)」と「$(“#セレクタ”).prop(“disabled”, true)」を使います。
<!DOCTYPE html>
<html lang="ja">
<head>
<script src="https://code.jquery.com/jquery-3.6.3.min.js" integrity="sha256-pvPw+upLPUjgMXY0G+8O0xUf+/Im1MZjXxxgOcBQBXU=" crossorigin="anonymous"></script>
<meta charset="UTF-8">
</head>
<body>
<p><button id="button">Button</button></p>
<p><select id="s1">
<option value="test1">test1</option>
<option value="test2">test2</option>
<option value="test3">test3</option>
</p>
<script>
$("#button").on("click", function(){
let s1_check = $("#s1").prop("disabled");
if(s1_check==true){
$("#s1").prop("disabled", false);
}else if(s1_check==false){
$("#s1").prop("disabled", true);
}
})
</script>
</body>
</html>
他にもチェックボックスやラジオボタンでも同じように選択可能か不可能かを変更することができると思います。
次回はブラウザで動くアプリを作るためのフレームワークStreamlitを紹介します。
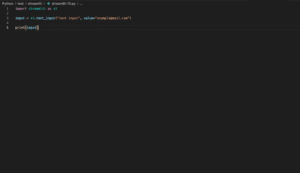
ではでは今回はこんな感じで。
コメント