NumPy
前回、Pythonで再帰処理を大量に行った場合に出る「RecursionError: maximum recursion depth exceeded while calling a Python object」の対処法を紹介しました。
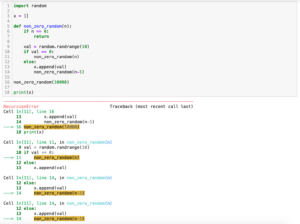
今回はNumPyでランダムな値を取得する方法を紹介します。
それでは始めていきましょう。
np.random.rand():0以上1未満のランダムな値
最初に紹介するのは「np.random.rand()」で、0以上1未満のランダムな値を取得できmす。
import numpy as np
print(np.random.rand())
実行結果
0.24715625765554183
これだけであれば「randomモジュール」の「random.random()」と変わりません。
NumPyのrandomモジュールでは、引数に数値を入れるとその数だけランダムな数値を得ることができます。
import numpy as np
print(np.random.rand(5))
実行結果
[0.70319587 0.1137922 0.44951358 0.05979427 0.40482892]
さらに引数を追加していくと、二次元配列、三次元配列としてランダムな値が得られます。
import numpy as np
print(np.random.rand(5, 3))
実行結果
[[0.40552336 0.25906295 0.31162809]
[0.60939871 0.21879683 0.16759167]
[0.2860627 0.98141914 0.93604207]
[0.60798239 0.52564058 0.01316258]
[0.25811018 0.83360434 0.67206372]]
import numpy as np
print(np.random.rand(5, 3, 4))
実行結果
[[[0.6409716 0.05279409 0.14760504 0.70597224]
[0.98809865 0.73339044 0.65842505 0.73017036]
[0.96426169 0.63433548 0.84526866 0.5822417 ]]
[[0.6744161 0.20481047 0.57810127 0.63296082]
[0.09883179 0.41920744 0.54064551 0.17478392]
[0.40430358 0.52952112 0.19381216 0.48731386]]
[[0.29669046 0.53861122 0.7525823 0.66412987]
[0.13266289 0.7218896 0.25044974 0.29229283]
[0.29629458 0.78557892 0.89336887 0.57187503]]
[[0.82445614 0.35029954 0.14105083 0.64249499]
[0.50816213 0.97131338 0.48093 0.77986018]
[0.20439653 0.87789543 0.6192995 0.83925949]]
[[0.27236964 0.28206113 0.36718157 0.19902706]
[0.835078 0.61413566 0.88329729 0.34752233]
[0.6793943 0.5565651 0.34032381 0.80337804]]]
np.random.randint():特定の範囲のランダムな値
「np.random.randint(a, b)」では「a以上、b未満」のなかの数値をランダムに取得します。
またこの場合、a、bは整数で、取得できる値も整数です。
import numpy as np
print(np.random.randint(3, 10))
実行結果
8
「np.random.randint()」では3つ目の引数にタプルで数値を渡すと、その数だけランダムに取得します。
import numpy as np
print(np.random.randint(3, 10, (5)))
実行結果
[5 5 6 5 8]
タプルの中に数値を追加していくと、二次元配列、三次元配列としてランダムに値を取得できます。
import numpy as np
print(np.random.randint(3, 10, (5, 3)))
実行結果
[[3 4 4]
[3 7 5]
[8 3 3]
[9 8 6]
[9 4 8]]
import numpy as np
print(np.random.randint(3, 10, (5, 3, 4)))
実行結果
[[[5 4 3 6]
[7 5 9 8]
[9 5 4 9]]
[[3 6 6 3]
[8 7 6 7]
[5 6 3 5]]
[[6 6 4 7]
[6 3 3 8]
[6 6 6 9]]
[[4 5 6 9]
[9 8 9 6]
[9 9 7 7]]
[[9 7 7 6]
[9 3 6 8]
[9 7 5 8]]]
np.random.randn():正規分布でランダムな値
「np.random.randn()」では正規分布となるランダムな値を取得できます。
import numpy as np
print(np.random.randn())
実行結果
-1.5775706047009612
引数に数値を与えるとその数だけ正規分布となるランダムな値を取得します。
import numpy as np
print(np.random.randn(5))
実行結果
[ 1.81706126 0.88176505 0.43736512 -0.82307079 -0.83155639]
さらに引数を追加すると、二次元配列、三次元配列として正規分布となるランダムな値を取得します。
import numpy as np
print(np.random.randn(5, 3))
実行結果
[[ 1.14133524 0.7885144 0.9371122 ]
[ 0.8581419 0.28322164 0.90834921]
[ 0.36871955 1.27203386 -0.2364113 ]
[-0.22197683 0.68539704 -0.36597064]
[ 0.52144118 1.44358382 -0.6697599 ]]
import numpy as np
print(np.random.randn(5, 3, 4))
実行結果
[[[-0.62432672 0.41123536 -0.66367759 -2.24440088]
[-0.91121522 1.19018423 -0.64085568 1.4992093 ]
[ 0.2981542 1.22230861 1.38514805 0.52134664]]
[[ 0.36631201 -1.70031374 -0.78325998 0.11254835]
[-0.53118519 -0.52987237 -0.44229857 0.78896318]
[ 2.33906538 0.44075248 1.02610293 -1.35428609]]
[[-0.61763683 1.31043768 -1.46095081 0.23868424]
[ 0.1725162 -0.31208571 -1.32273264 -1.50720934]
[ 0.71890781 -0.47967986 1.34117949 -0.54460227]]
[[-0.27338127 1.03133741 0.82767241 -0.61217282]
[-1.87651475 0.10912745 1.41675278 0.37382911]
[-0.60684055 0.05084218 0.36252401 -0.08591229]]
[[-0.82637849 0.7680066 0.98352023 0.62704024]
[-0.07489152 -0.89456613 0.74616685 -1.53731337]
[ 1.29539137 0.79241883 -1.56339558 -1.11852751]]]
おまけとしてこの取得した値が本当に正規分布に沿っているのか確認してみました。
import matplotlib.pyplot as plt
import numpy as np
random_data = [np.random.randn() for _ in range(10000)]
def gauss(x_list, a=1, m=0, s=1):
y_list = [a * np.exp(-(x - m)**2 / (2*s**2)) for x in x_list]
return y_list
x_list = np.arange(-5, 5, 0.1)
y_list = gauss(x_list)
fig = plt.figure()
plt.clf()
ax1 = fig.subplots()
ax2 = ax1.twinx()
ax1.hist(random_data, bins=50, color="tab:blue")
ax2.plot(x_list, y_list, color="tab:orange")
ax2.set_ylim(0,1.1)
plt.show()
実行結果

確かに正規分布に沿っているようです。
ちなみに二項分布「numpy.random.binomial()」、ベータ分布「numpy.random.beta()」、ガンマ分布「numpy.random.gamma()」、カイ二乗分布「numpy.random.chisquare()」という分布に関してもランダムな値を取得できるようです。
次回はNumPyで全ての要素が0の配列を作成する方法を紹介します。
ではでは今回はこんな感じで。
コメント