tweepy
前回、LINE Notify APIを使ってメッセージを自分のアカウントにメッセージを送る方法を解説しました。
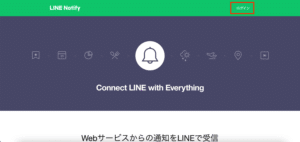
そして今回は前々回に作成した「自分のタイムラインから条件に合う未フォローのアカウントを抽出」するプログラムにこのLINE Notify APIでのメッセージ送信の機能を追加していきたいと思います。
ちなみに前に作成したプログラムはこんな感じ。
import tweepy
follower_min = 10000
ff_ratio_min = 0.9
ff_ratio_max = 1.2
account = "@account_name"
consumer_key = ' consumer key '
consumer_secret = ' consumer secret '
access_token = ' access token '
access_token_secret = ' access token secret '
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth, wait_on_rate_limit = True)
def gettimeline():
tweets = tweepy.Cursor(api.home_timeline, id=account).items(200)
return tweets
def getscreenname(tweets):
collected_users = []
for tw in tweets:
if not tw.user.screen_name == account.lstrip('@'):
if tw.text.startswith("RT"):
user = tw.retweeted_status.user.screen_name
followers = tw.retweeted_status.user.followers_count
follows = tw.retweeted_status.user.friends_count
following = tw.retweeted_status.user.following
if following == False:
if follows == 0:
follows = 1
if followers >= follower_min and ff_ratio_min <= followers/follows <= ff_ratio_max:
if not user in collected_users:
collected_users.append(f"{user}")
return collected_users
def main():
tweets = gettimeline()
collected_users = getscreenname(tweets)
print(collected_users)
if __name__ == '__main__':
main()
それでは始めていきましょう。
設定部分への追加
まずは設定部分にLINE Notify APIの情報を追加していきます。
ということでこんな感じ。
import tweepy
import requests
follower_min = 10000
ff_ratio_min = 0.9
ff_ratio_max = 1.2
account = "@account_name"
consumer_key = ' consumer key '
consumer_secret = ' consumer secret '
access_token = ' access token '
access_token_secret = ' access token secret '
auth = tweepy.OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
api = tweepy.API(auth, wait_on_rate_limit = True)
line_notify_token = ' 取得したアクセストークン '
line_notify_api = 'https://notify-api.line.me/api/notify'
変更部分としては、requestsのインポートの追加と最後にLINE Notify APIのアクセストークンとアクセスするURLの追加です。
自作関数部分
自作関数として「gettimeline」、「getscreenname」がありますが、これはそのままで大丈夫です。
今回はさらに「sendlinemessage」という取得したアカウント名を送るための関数を追加します。
def sendlinemessage(collected_users):
headers = {'Authorization': f'Bearer {line_notify_token}'}
message = ''
for user in collected_users:
user_url = f'https://twitter.com/{user}'
message = message + user_url + '\n'
data = {'message': message}
requests.post(line_notify_api, headers = headers, data = data)
headersに関しては前回解説しているので、今回は説明を割愛します。
ただ送信するメッセージに関しては、取得したアカウント名からワンタップでTwitterに接続して、アカウントを表示できるように細工をしていきます。
message = ''
for user in collected_users:
user_url = f'https://twitter.com/{user}'
message = message + user_url + '\n'
この部分でやっていることとしては抽出したアカウント名のリストからfor文を使って一つずつアカウント名を取り出します。
そしてワンタップでアクセスできるようにするためTwitterのユーザープロフィールのURL(https://twitter.com/ユーザー名)にします。
そして作成したURLを変数messageに繋げていくことによって、送信するメッセージとします。
あとはURLが詰め込まれた変数messageを辞書dataに格納し、requestsでLINE Notify APIにpostします。
data = {'message': message}
requests.post(line_notify_api, headers = headers, data = data)
これで自作関数部分の変更は完了です。
main関数
次にmain関数部分を修正します。
def main():
tweets = gettimeline()
collected_users = getscreenname(tweets)
sendlinemessage(collected_users)
修正としては、「print(collected_users)」として抽出したアカウント名を表示していた部分を削除し、先ほどの「sendlinemessage関数」を追加しただけです。
これで実行してみると、こんな感じで抽出したアカウントへのリンクがLINEで受信できました。
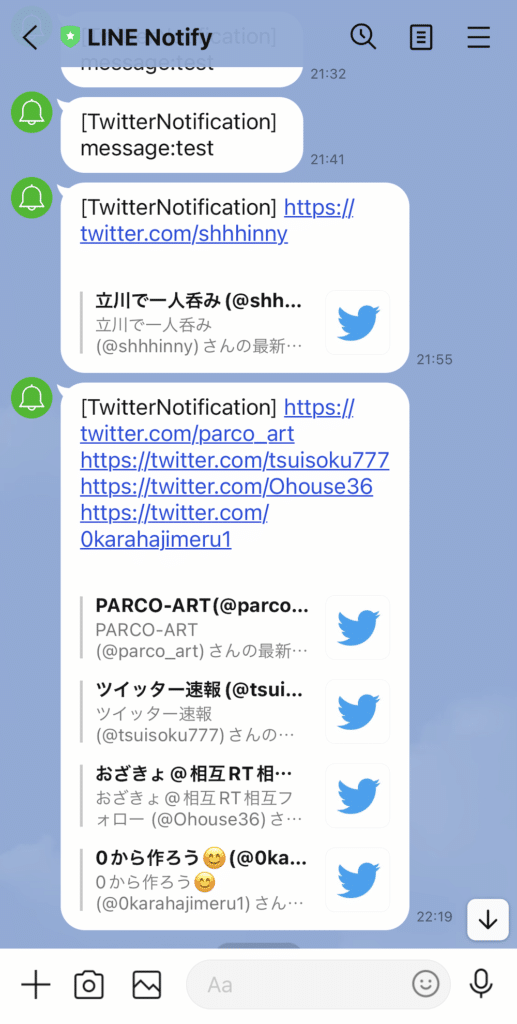
これでフォロワー探索がだいぶ効率的になることでしょう。
よかったら皆さんも試してみてください。
次回はTwitter APIとLINE Notify APIを使って、Twitterで検索をかけた上、特定の条件に当てはまるツイートをLINEに通知するということをやってみます。
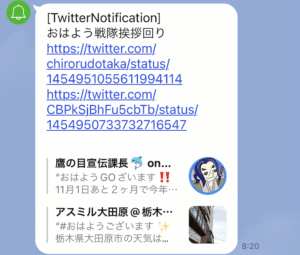
ではでは今回はこんな感じで。
コメント