Twitter API v2
前回、TweepyからTwitter API v2を使い、ユーザーID、タイムライン、ツイートの取得を行いました。
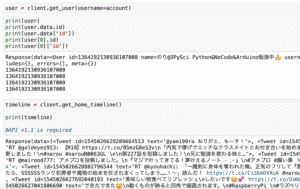
今回はフォロー、フォロワーの取得を行なっていきます。
まずはAPIへの接続ですが、最初はとりあえずTwitter API v2にだけ接続しますので、Bearer Tokenだけを使用します。
import tweepy
account = 'Your username'
BT = "Your bearer token"
client = tweepy.Client(bearer_token=BT)
また前回学んだようにTwitter API v2ではユーザーIDをよく使いますので自作関数を用意しました。
def userIdGet(account):
user = client.get_user(username=account)
return user[0]['id']
accountid = userIdGet(account)
print(accountid)
それでは始めていきますが、ここからは私のアカウント(@3PySci_Nori)を例とって解説していきます。
フォロワーの取得
まずはフォロワーを取得してみます。
フォロワー取得には「.get_users_followers(ユーザーID)」を使用します。
followers = client.get_users_followers(accountid)
print(followers)
実行結果
Response(data=[<User id=1541575623449141248 name=さな💮Webコーチング username=Web41917654>,
<User id=1525007479112814592 name=バレンティノ藤田 username=dsfYhepFqEDZIA7>,
<User id=1254962436 name=Mann Pugh username=PughMann>,
<User id=1544545760783781888 name=マジでサッカー好きなんすよ!!宣伝垢 username=tXyKSr53JUgGDkx>,
(中略)
<User id=1532299246178684928 name=信長FX『1万円から始められるFX自動売買【金乱舞】』 username=okutorex4>,
<User id=1342815448920858624 name=星奈実沙希🪐🧊蓋然性オルトイズム username=_misaki_world>,
<User id=1511865558425550851 name=中野若菜@HEROZ㍿ 採用担当 username=t7TMe6C>,
<User id=1537428315518054404 name=Runa🍓脱貧乏 username=Runa67565177>,
<User id=1361875602328887298 name=⏹️世界絶景⏹️ username=zekkei7771>],
includes={}, errors=[], meta={'result_count': 100, 'next_token': 'EV80DF62IOBHGZZZ'})
フォロワーのIDやユーザーネームに関しては「data」の中に入っています。
そのため数を取得できたフォロワー数を数えるのはこんな感じです。
print(len(followers.data))
実行結果
100
また特定の人のユーザーIDを取得するにはその人のインデックス番号を指定し、「.get(‘id’)」とします。
print(followers.data[0].get('id'))
一度に取得できる数はデフォルトで100人で、前回のツイートを取得した際と同様、「next token」を使うことで、さらにフォロワーを取得できます。
followers = []; pagination_token = None
while len(followers) < 300:
follower = client.get_users_followers(accountid, pagination_token=pagination_token)
for user in follower[0]:
followers.append(user)
pagination_token = follower[3]['next_token']
print(len(followers))
実行結果
300
フォローしている人の取得
次にフォローしている人を取得してみましょう。
フォローしている人を取得するには「.get_users_following(ユーザーID)」を使用します。
そのためページネーションを使って、一度に100人以上のフォローしている人を取得するのはこんな感じです。
followeds = []; pagination_token = None
while len(followeds) < 300:
followed = client.get_users_following(accountid, pagination_token=pagination_token)
for user in followed[0]:
followeds.append(user)
pagination_token = followed[3]['next_token']
print(len(followeds))
実行結果
300
自分がフォローしているか確認
ついでに自分がフォローしているのか、もしくはフォローされているのかIDから知りたくなってきます。
しかし残念ながらこれらはTwitter API v2ではまだ機能が実装されていないようです(2022年7月)。
またTwitter API v1.1では自分がフォローしているかどうかは分かりますが、フォローされているかどうかは分からないようです。
とりあえず現状できる「自分がフォローしているかどうかの確認」方法を紹介しておきます。
ここからはTwitter API v1.1に接続します。
auth = tweepy.OAuthHandler('Your API Key', 'Your API Secret')
auth.set_access_token('Your Access Token', 'Your Access Token Secret)
api = tweepy.API(auth)
特定のユーザーの情報を取得する「.get_user()」
user = api.get_user(user_id=1434687719490211842)
print(user)
実行結果
User(_api=<tweepy.api.API object at 0x10e58a080>, _json={'id': 1434687719490211842, 'id_str':
'1434687719490211842', 'name': '漢字間違い探し', 'screen_name': 'FindDiffKanji', 'location': '',
'profile_location': None, 'description': '埋め尽くされた #漢字 の中に一つだけ、違う漢字が含まれています。
貴方はその漢字を見つけ出すことができますか? #クイズ #間違い探し #脳トレ
(中略)
ill_color='DDEEF6', profile_text_color='333333', profile_use_background_image=True,
has_extended_profile=True, default_profile=True, default_profile_image=False, following=True,
follow_request_sent=False, notifications=False, translator_type='none', withheld_in_countries=[])
この中の「following」という項目が自分がフォローしているかどうかの項目です。
取得するにはこのようにします。
print(user.following)
実行結果
True
ということでフォロー、フォロワーが取得できるようになりました。
次回はアカウントIDを取得するのに使っている「get_user」でさらに詳細な情報を取得してみましょう。
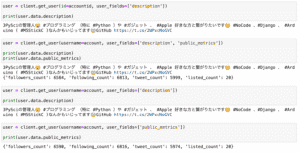
ではでは今回はこんな感じで。
コメント